In our last post, we discussed how to save an image – as a binary large object, or blob – into a mySQL database using PHP.
In this followup, we’ll be putting that image to actual use as we retrieve it from the database and display it.
The code required to get the image into the database was fairly complex, but it also did most of the overall work that needs to be done to the image. In fact, to display the image (like the book cover paired with a title used in our last post), all we really need is a small block of PHP code:
<?php
require_once "config.php";
$conn = new mysqli(DB_SERVER, DB_USERNAME, DB_PASSWORD, DB_NAME);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
else {
$sql = "SELECT * FROM books ORDER BY title";
$results = mysqli_query($conn, $sql);
while ($book = mysqli_fetch_assoc($results)) {
echo '<fieldset style="width: 300px;"><legend>' .$book["Title"]. '</legend><a href="viewer.php? id=' .$book['_pkey']. '" ><img class="coverImage" src="data:image/png;base64,' .base64_encode($book["Cover"]). '" style="width: 300px;"></a></fieldset><br><br>';
}
}
?>
(the require_once of config.php is just including out helper file that stores the database credentials)
The code can be a little bit dense, but the main things to know are that the img src sets the data type to a base64 .png, then echoes out to make the actual src a base64-encoded version of the cover stored in the database.
(The while loop, meanwhile, simply iterates through each of the rows until none remain, in order to display all of them on the page)
To summarize, this is the main part you need to pay attention to:
src="data:image/png;base64,' .base64_encode($book["Cover"]).
And, if you’ve followed the steps (or the principles) outlined in the previous post and this one, you should have something like this!
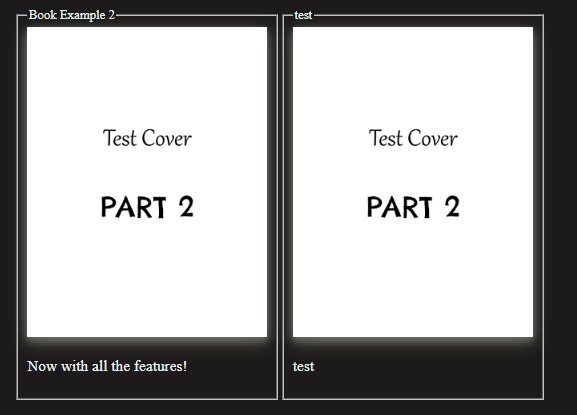
Next up, we’ll be adding some extra functionality – previewing the image on the upload page before adding it to the database!