Recently, I was working on a personal project that, due to its nature, was best served by saving a handful of images in a mySQL database along with my other data. I spent quite a while researching how to do so, and mostly found articles saying not to do it; to simply save the image to the filesystem and link to it in the database instead.
But I’m stubborn.
While that may be the best usage for most cases, I wanted to figure out how to do the thing. It took some doing, but I eventually found the answer.
Saving an Image to a mySQL Database using PHP
First off, let’s set up the form that we’ll be interacting with. In this example, we’ll be uploading the title of a book and a cover for it. Note the “enctype=”multipart/form-data” – that’s one part of the puzzle for getting this to work correctly. That’s what lets the browser know not to encode any of the data, since that will mess up our file upload.
<form method="post" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>" enctype="multipart/form-data">
Title: <input id="bookTitle" name="bookTitle" type="text" size="20" required value="<?php echo $title;?>"><br><br>
Cover: <input type="file" name="cover" id="cover" accept=".png" onchange="readURL(this)"><br><br>
<input type="submit" value="Submit" id="Submit"><br><br>
</form>
Next up, we look at what happens in the PHP code when the form posts:
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$title = $_POST["bookTitle"];
$cover = addslashes(file_get_contents($_FILES["cover"]["tmp_name"]));
$conn = new mysqli(DB_SERVER, DB_USERNAME, DB_PASSWORD, DB_NAME);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
else {
$sql = "INSERT INTO books (title, cover) VALUES ('{$title}', '{$cover}')"; }
We set our variables – $title and $cover – and in so doing we use the functions that will prepare our image file to be properly encoded. The file_get_contents() reads the file itself into a string, then the addslashes() returns a string with backslashes in front of predefined characters – the special characters that would otherwise cause us problems during the database insert.
After that we make our mySQL connection (using a helper file I had previously set up to handle credentials), and if the connection doesn’t fail we make our query, etc.
(make sure that you have set the column for your image’s type as a blob – a binary large object. This will enable the database to handle the insertion.)
All that done, you should have a working form that will take an image uploaded to the page – this one specifically only accepts .png files – and insert it into the database. Here’s what mine looks like, though mine has some extra features, such as an image preview.
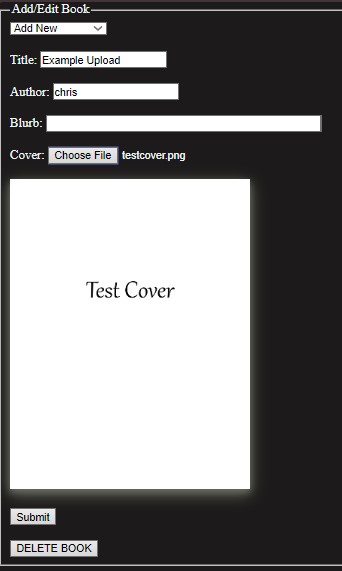
And there you have it! an image in a database – what many said can’t (or at least should never, which I disagree with) be done.
In the next post, we’ll discuss how to retrieve and display the image you just uploaded!